API Documentation¶
Our RESTful [1] API allows you send text messages directly from your app. With our API you can:
- Send a text message
- Check your account balance
- Top up account balance
- Check the delivery status of a message
- Retrieve contacts saved in your account
How It Works¶
- Your application sends a request to SimpleSell SMS requesting to have a text message sent
- SimpleSell SMS acknowledges receipt of the message with a success or error message. An error message may be generated if there is something wrong with the format or contents of the request or if you have insufficient tokens to complete the request
- If the target phone number is missing from your contacts list on the SimpleSell SMS dashboard, the contact is added to our contacts list
- The text message is forwarded to our SMS gateway for sending
- SimpleSell SMS sends back delivery status information to your application (you need to provide a callback URL for this)
Gettings Started¶
You will need to log into your account and enable the API feature. Once you are logged in, click on the My Account menu (the one showing your name) that is displayed on the top right hand side of any page. Click on the Account Settings option.
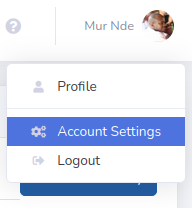
Account Settings Menu
You will be presented with a form like the one below to configure various options.
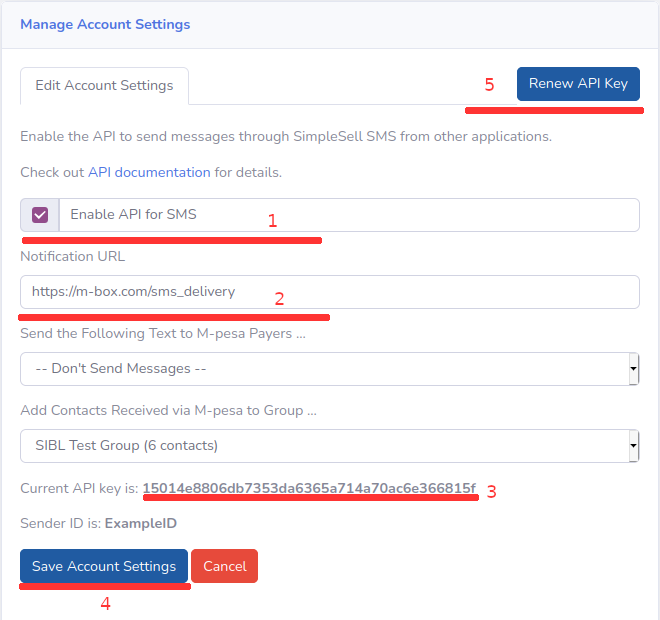
Manage Account Settings
The labelled items are as follows:
- Enable/disable API: If checked, then API is enabled
- Notification URL: SMS delivery notifications will be sent to this address. This is optional
- Current API Key: You will use this key as a way to authenticate yourself when making an API request
- Save Settings: Click here to save any changes you’ve made
- Renew API Key: If your current API key has been exposed or you believe for whatever reason that it’s been compromised, click here to generate a new key and invalidate the current one
How to Send Messages via the API¶
Sending a Single SMS¶
To send an SMS, do a HTTP POST
request to the end point https://sms.simplesellable.com/api/v1/send
The request should be structured as follows:
{
"senderid": "ExampleID",
"country": "KE",
"timestamp": 1568826519,
"verification": "c4495df2f6d7de8bef689ff85551cfee880cf994",
"mobile": "254721234567",
"sms": "Your insurance for ABC Life is due on 20/03/2020. Amount due is KES2,500. Visit our website to pay.",
"timetosend": 1568826519,
"name": {
"title":"Dr",
"fname":"John",
"mname":"Michael",
"lname":"Doe"
}
}
The parameters correspond to the following information:
- senderid: the sender ID registered to your account for sending out messages
- country: 2 letter ISO code for the country the senderid is registered in
- timestamp: the current Unix/UTC timestamp (should be an integer value. 5 minutes margin of error allowed)
- verification: a code to authenticate the request. This is calculated as follows:
sha1(timestamp + api_key)
(SHA1 hash of the concatenation of timestamp and API key) - mobile: the mobile number of the recipient, starting with country code. Only digits are allowed
- sms: the text message to send. If the message is being sent to a first time recipient, an unsubscribe notice (see How to Unsubscribe) will be appended to the end of the message and this may affect cost
- timetosend: this is an optional Unix/UTC timestamp that may be used to queue a message for sending later. If it’s not provided or is set to a time in the past, the message will be sent immediately
- name: this optional parameter is an object consisting of the title/salutation (title), first name (fname), middle name (mname) and last name (lname) of the recipient. It will be used in creating a contact if the recipient is not in your contacts list already. Should you choose to use this parameter, only fname is required. If this parameter is not provided and the recipient is not in your contacts list, the recipient name will be saved as Unknown
Important
The timestamp on the API request cannot be older than 5 minutes. Any request older than 5 minutes will be rejected.
Once your request has been submitted successfully, you will receive a response in the following format:
{
"status": {
"code": 0,
"message": "Message queued successfully",
"tokens": 1,
"smsid": 22905
}
}
The meaning of the items in the response are as follows:
- code: indicates whether the action was successfull, with 0 being success and any other value being failure
- message: an explanation for the code above
- tokens: number of tokens consumed by the request
- smsid: a unique identifier for the message sent out
Sending to Multiple Contacts at Once¶
If you need to send the same message to multiple contacts, the batch SMS endpoint is the one to use. To send an SMS, do a HTTP POST
request to the end point https://sms.simplesellable.com/api/v1/send/batch
The request should be structured as follows:
{
"senderid": "ExampleID",
"country": "KE",
"timestamp": 1568826519,
"verification": "c4495df2f6d7de8bef689ff85551cfee880cf994",
"sms": "Hi [first name]. You internet bill of KES[amount] is due on [deadline]. Please pay before then to avoid service disruption.",
"timetosend": 1568826519,
"contacts": [
{
"title":"Prof",
"fname":"John",
"mname":"Michael",
"lname":"Doe",
"mobile": "254721234567",
"extra": [
{
"deadline": "2nd Jan 2021"
},
{
"amount": "1,500"
}
]
},
{
"title":"Ms",
"fname":"Jane",
"mname":"Wanjiru",
"lname":"Gitaka",
"mobile": "254719554670",
"extra": [
{
"deadline": "4nd Feb 2021"
},
{
"amount": "2,360"
}
]
}
]
}
The parameters correspond to the following information:
senderid: the sender ID registered to your account for sending out messages
country: 2 letter ISO code for the country the senderid is registered in
timestamp: the current Unix/UTC timestamp (should be an integer value. 5 minutes margin of error allowed)
verification: a code to authenticate the request. This is calculated as follows:
sha1(timestamp + api_key)
(SHA1 hash of the concatenation of timestamp and API key)sms: the text message to send. If the message is being sent to a first time recipient, an unsubscribe notice (see How to Unsubscribe) will be appended to the end of the message and this may affect cost
timetosend: this is an optional Unix/UTC timestamp that may be used to queue a message for sending later. If it’s not provided or is set to a time in the past, the message will be sent immediately
contacts: this is an array of recipient contact information. The same message will be sent to each recipient and can be customised with information such as each recipient’s name. Each contact has the following information:
- title: the title or salutation of the recipient. This is an optional parameter
- fname: the first name of the recipient. If this parameter is not provided and the recipient is not in your contacts list, the recipient name will be saved as Unknown
- mname: middle name of the recipient. This is an optional parameter
- lname: last name of the recipient. This is an optional parameter
- mobile: the mobile number of the recipient, starting with country code. This is required. Only digits are allowed
- extra: this optional parameter allows you to include custom fields in the SMS. This can be information such as the amount the recipient owes, invoice number, account number, date due etc. Each custom field must be an array associative array. To insert this field into the SMS, wrap its name in square brackets e.g. [amount] for amount field.
Once your request has been submitted successfully, you will receive a response in the following format:
{
"status": {
"code": 0,
"message": "Message queued successfully",
"tokens": 2,
"smsids": [
22905,
22906
]
}
}
The meaning of the items in the response are as follows:
- code: indicates whether the action was successfull, with 0 being success and any other value being failure
- message: an explanation for the code above
- tokens: number of tokens consumed by the request
- smsids: an array with unique identifiers for each message sent out
Creating an SMS Campaign¶
You can send a message to a group defined on the SimpleSell SMS online platform using the campaign API endpoint. Besides being more efficient, this allows you to log into the online dashboard and get reports on the campaign. You can learn more about SMS campaigns here: How to Message a Group (SMS Campaign)
To create a campaign, do a HTTP POST
request to the end point https://sms.simplesellable.com/api/v1/send/campaign
The request should be structured as follows:
{
"senderid": "ExampleID",
"country": "KE",
"timestamp": 1568826519,
"verification": "c4495df2f6d7de8bef689ff85551cfee880cf994",
"sms": "Hi [first name]. We will be performing maintenance from 3.00am to 5.00am on Sunday. During this time you might experience intermittent service. Please bear with us.",
"groupid": 34,
"timetosend": 1568826519
}
The parameters correspond to the following information:
- senderid: the sender ID registered to your account for sending out messages
- country: 2 letter ISO code for the country the senderid is registered in
- timestamp: the current Unix/UTC timestamp (should be an integer value. 5 minutes margin of error allowed)
- verification: a code to authenticate the request. This is calculated as follows:
sha1(timestamp + api_key)
(SHA1 hash of the concatenation of timestamp and API key) - sms: the text message to send. If the message is being sent to a first time recipient, an unsubscribe notice (see How to Unsubscribe) will be appended to the end of the message and this may affect cost
- groupid: this is the target group for the campaign. See Retrieve Groups to learn how to retieve a list of groups.
- timetosend: this is an optional Unix/UTC timestamp that may be used to queue a campaign for sending later. If it’s not provided or is set to a time in the past, the campaign will be processed and sent immediately
Once your request has been submitted successfully, you will receive a response in the following format:
{
"status": {
"code": 0,
"message": "Campaign with 17 messages created and queued successfully",
"tokens": 17,
"smsids": [
22998,
22999,
23000,
23001,
23002,
23003,
23004,
23005,
23006,
23007,
23008,
23009,
23010,
23011,
23012,
23013,
23014
]
}
}
The meaning of the items in the response are as follows:
- code: indicates whether the action was successfull, with 0 being success and any other value being failure
- message: an explanation for the code above
- tokens: number of tokens consumed by the request
- smsids: an array with unique identifiers for each message sent out
API Response Codes¶
The following are the various responses you can expect from the API when sending a message:
Code | Meaning |
---|---|
0 | Success |
1 | Invalid request method (e.g. GET instead of POST) |
2 | Missing parameter |
3 | Invalid timestamp |
4 | Invalid parameter value |
5 | Insufficient balance (top up your account) |
6 | Request failed |
Getting Delivery Acknowledgements¶
Delivery status notifications will be sent to the URL address provided in the Manage Account Settings page. The response will be structured as follows:
{
"smsid": 22905,
"tokens": 1,
"status": "Sent"
}
The meaning of the items in the response is as follows:
- smsid: a unique identifier corresponding to the message sent out earlier
- tokens: number of tokens consumed by sending out the message
- status: message delivery status. See SMS Delivery Status for more information
How to Check Account Balance via API¶
To check your account balance, do a HTTP POST
request to the end point https://sms.simplesellable.com/api/v1/balance
The request should be structured as follows:
{
"senderid": "ExampleID",
"country": "KE",
"timestamp": 1585329158,
"verification": "4c2a4f6a7d1f269a7ab3ec99f05254889883a370"
}
The parameters correspond to the following information:
- senderid: the sender ID registered to your account for sending out messages
- country: 2 letter ISO code for the country the senderid is registered in
- timestamp: the current Unix/UTC timestamp (should be an integer value. 5 minutes margin of error allowed)
- verification: a code to authenticate the request. This is calculated as follows:
sha1(timestamp + api_key)
(SHA1 hash of the concatenation of timestamp and API key)
Once your request has been submitted successfully, you will receive a response in the following format:
{
"status": {
"code": 0,
"message": "Balance queried successfully",
"tokens": 134,
"dedicated": 0
}
}
The tokens field will indicate your account balance in tokens.
The dedicated field will indicate whether the account is using a dedicated or a shared SMS sender ID.
For the meaning of the various response codes please refer to API Response Codes.
How to Top Up Account via API¶
You can add credits to your account via M-pesa Online Checkout. You will need to enter your mobile money PIN on your handset to complete payment. To initiate online checkout via the API, do a HTTP POST
request to the end point https://sms.simplesellable.com/api/v1/topup
The request should be structured as follows:
{
"senderid": "ExampleID",
"country": "KE",
"timestamp": 1585329158,
"verification": "4c2a4f6a7d1f269a7ab3ec99f05254889883a370",
"mobile": "254721234567",
"amount": 500
}
The parameters correspond to the following information:
- senderid: the sender ID registered to your account for sending out messages
- country: 2 letter ISO code for the country the senderid is registered in
- timestamp: the current Unix/UTC timestamp (should be an integer value. 5 minutes margin of error allowed)
- verification: a code to authenticate the request. This is calculated as follows:
sha1(timestamp + api_key)
(SHA1 hash of the concatenation of timestamp and API key) - mobile: the mobile number of the recipient, starting with country code. Only digits are allowed
- amount: the amount to top up with. Should be an integer – only digits are allowed
Once your request has been submitted successfully, you will receive a response in the following format:
{
"status": {
"code": 0,
"message": "Payment request initiated successfully",
"tokens": 500
}
}
The tokens field will indicate the number of tokens you’ll recieve if your payment is success.
For the meaning of the various response codes please refer to API Response Codes.
Important
You can use the account balance API endpoint to confirm that your topup was successful.
How to Check SMS Delivery Status¶
When you send an SMS via the API, the delivery status is sent to the URL address you provided when configuring the API. If however the delivery status was not received, you can check the delivery status via our status
API by sending a HTTP POST
request to https://sms.simplesellable.com/api/v1/status
.
The request should be structured as follows:
{
"senderid": "ExampleID",
"country": "KE",
"timestamp": 1585329158,
"verification": "4c2a4f6a7d1f269a7ab3ec99f05254889883a370",
"smsid": 22905
}
The parameters correspond to the following information:
- senderid: the sender ID registered to your account for sending out messages
- country: 2 letter ISO code for the country the senderid is registered in
- timestamp: the current Unix/UTC timestamp (should be an integer value. 5 minutes margin of error allowed)
- verification: a code to authenticate the request. This is calculated as follows:
sha1(timestamp + api_key)
(SHA1 hash of the concatenation of timestamp and API key) - smsid: a unique identifier corresponding to the message sent out earlier via the API
Once your request has been submitted successfully, you will receive a response in the following format:
{
"status": {
"code": 0,
"message": "Status queried successfully",
"smsid": 22905,
"tokens": 1,
"status": "Sent"
}
}
The meaning of the items in the response is as follows:
- code: indicates whether the action was successfull, with 0 being success and any other value being failure
- message: an explanation for the code above
- smsid: a unique identifier corresponding to the message sent out earlier
- tokens: number of tokens consumed by sending out the message
- status: message delivery status. See SMS Delivery Status for more information
Important
You can only check the status of text messages sent via the API and not those sent via the dashboard. The status information is only available for one week from the date and time of sending.
How to Retrieve Contacts¶
Retrieve Groups¶
All contacts on SimpleSell SMS are organised in groups (see Managing Groups to learn more). To retrieve an account’s list of groups, send a HTTP POST
request to https://sms.simplesellable.com/api/v1/contacts/groups
The request should be structured as follows:
{
"senderid": "ExampleID",
"country": "KE",
"timestamp": 1612301372,
"verification": "51c57f51857c52655784c8d8d7f7aaca9b00425a",
"startat": 0,
"groupid": 23
}
The parameters correspond to the following information:
- senderid: the sender ID registered to your account for sending out messages
- country: 2 letter ISO code for the country the senderid is registered in
- timestamp: the current Unix/UTC timestamp (should be an integer value. 5 minutes margin of error allowed)
- verification: a code to authenticate the request. This is calculated as follows:
sha1(timestamp + api_key)
(SHA1 hash of the concatenation of timestamp and API key) - startat: an optional parameter indicating the number of records to skip. The default is zero, meaning do not skip any records. This is useful if you have more than 100 contact and need to retrieve records after the 100th one
- groupid: an optional parameter. Use this to retrieve information on a specific group only
Once your request has been submitted successfully, you will receive a response in the following format:
{
"status": {
"code": 0,
"message": "Group information retrieved successfully",
"tokens": 0,
"startat": 0,
"totalgroups": 4,
"groups": {
"28": {
"name": "All Contacts",
"description": "Default Group",
"totalcontacts": "112",
"groupid": "28"
},
"12": {
"name": "Murang'a Agents",
"description": "Murang'a Agents",
"totalcontacts": "17",
"groupid": "12"
},
"13": {
"name": "Nyeri Agents",
"description": "Nyeri Agents",
"totalcontacts": "76",
"groupid": "13"
},
"15": {
"name": "Kiambu Agents",
"description": "Kiambu Agents",
"totalcontacts": "92",
"groupid": "15"
}
}
}
}
The meaning of the items in the response is as follows:
- code: indicates whether the action was successfull, with 0 being success and any other value being failure
- message: an explanation for the code above
- tokens: number of tokens if any consumed by the request
- startat: number of records skipped if any
- totalgroups: total number of group records in the account
- groups: an array with all the records retrived. Each record has the following information:
- name: name of the group
- description: explanation (as provided by user) of what the group is about
- totalcontacts: total contacts in the group
- groupid: unique identifier of the group
Retrieve Individual Contacts¶
You can retrieve contacts from your online address book via the API in batches of up to 100 contacts. To do this, send a HTTP POST
request to https://sms.simplesellable.com/api/v1/contacts
The request should be structured as follows:
{
"senderid": "ExampleID",
"country": "KE",
"timestamp": 1585329158,
"verification": "4c2a4f6a7d1f269a7ab3ec99f05254889883a370",
"startat": 100,
"groupid": 23,
"mobile": 254721234567,
"unsubscribed": 1,
"modifiedafter": 1568658219
}
The parameters correspond to the following information:
- senderid: the sender ID registered to your account for sending out messages
- country: 2 letter ISO code for the country the senderid is registered in
- timestamp: the current Unix/UTC timestamp (should be an integer value. 5 minutes margin of error allowed)
- verification: a code to authenticate the request. This is calculated as follows:
sha1(timestamp + api_key)
(SHA1 hash of the concatenation of timestamp and API key) - startat: an optional parameter indicating the number of records to skip. The default is zero, meaning do not skip any records. This is useful if you have more than 100 contact and need to retrieve records after the 100th one
- groupid: an optional parameter. Use this to retrieve contacts from a specific group
- mobile: an optional parameter. Use this to retrieve information for a specific contact (retrieve a single record)
- unsubscribed: an optional parameter. Use this to choose whether to retrieve subscribed contacts only (value of 0) or unsubscribed contacts only (value of 1). Default value is 0
- modifiedafter: an optional parameter. This is a Unix/UTC timestamp. Use this to only retrieve records created or modified after a specified time. Useful for retrieving modified and new contacts
Once your request has been submitted successfully, you will receive a response in the following format:
{
"status": {
"code": 0,
"message": "Contact information retrieved successfully",
"tokens": 0,
"startat": 100,
"totalcontacts": 102,
"contacts": {
"254721234567": {
"title": "Mrs",
"name": "Mary Ann Thuku",
"fname": "Mary",
"mname": "Ann",
"lname": "Thuku",
"country": "KE",
"mobile": "254721234567",
"lastmodified": 1568658219,
"unsubscribed": "0"
},
"254714655811": {
"title":"Rev",
"name":"Fundi Konde Nkruma",
"fname":"Fundi",
"mname":"Konde",
"lname":"Nkruman",
"country":"KE",
"mobile": "254714655811",
"lastmodified": 1568658219,
"unsubscribed": "0"
},
}
}
}
The meaning of the items in the response is as follows:
- code: indicates whether the action was successfull, with 0 being success and any other value being failure
- message: an explanation for the code above
- tokens: number of tokens if any consumed by the request
- startat: number of records skipped if any
- totalcontacts: total number of contact records in the account
- contacts: an array with all the records retrived. Each record has the following information:
- title: title or salutation of contact
- name: full name of contact
- fname: first name of contact
- mname: middle name of contact
- lname: last name of contact
- country: 2 letter ISO code of the country the phone number is registered in
- mobile: the mobile number of the contact with country code
- lastmodified: a Unix/UTC timestamp indicating when the contact was last modified or created (if never modified)
- unsubscribed: whether the contact has opted out of receiving text messages or not. If the value is 1, then messages to this contact will not be delivered
Footnotes
[1] | The acronym REST stands for REpresentational State Transfer. This means that each unique URL is a representation of some object. |